Contrariwise, continued Tweedledee, if it was so, it might be, and if it were so, it would be; but as it isn’t, it ain’t. That’s logic!
TweedleDee
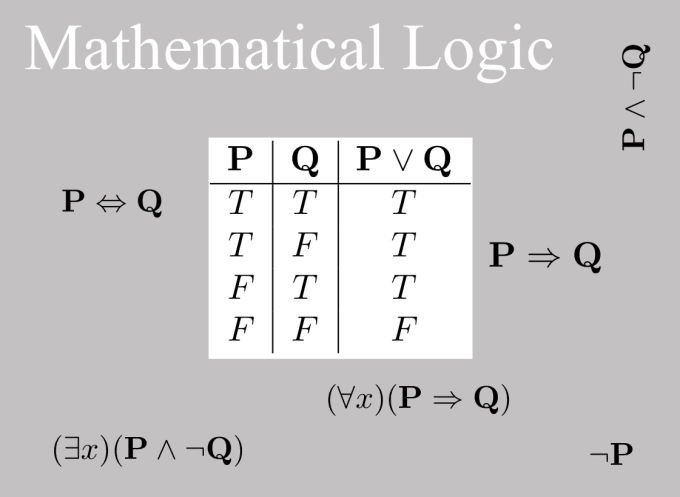
First, i trust everyone is safe.
Second, i am going to be pushing a blog out every Wednesday called Snake_Bytes. This is the second one hot off the press. Snake as in Python and Bytes as in well you get it. Yes, it is a bad pun but hey most are bad.
i will pick one of the myriads of python based books i have in my library and randomly open it to a page. No matter how basic or advanced i will start from there and i will create a short concise blog on said subject. For some possibly many the content will be rather pedantic for others i hope you gain a little insight. As a former professor told me “to know a subject in many ways is to know it well.” Just like martial arts or music performing the basics hopefully makes everything else effortless at some point.
Ok so in today’s installment we have Comparison and Equality.
I suppose more philosophically what is the Truth?
All Python objects at some level respond to some form of comparisons such as a test for equality or a magnitude comparison or even binary TRUE
and FALSE
.
For all comparisons in Python, the language traverses all parts of compound objects until a result can be ascertained and this includes nested objects and data structures. The traversal for data structures is applied recursively from left to right.
So let us jump into some simple snippets there starting with lists objects.
List objects compare all of their components automatically.
%system #command line majik in Jupyterlab
# same value with unique objects
A1 = [2, (‘b’, 3)]
A2 = [2, (‘b’, 3)]
#Are they equivalent? Same Objects?
A1 == A2, A1 is A2
(True, False)
So what happened here? A1
and A2
are assigned lists which in fact are equivalent but distinct objects.
So for comparisons how does that work?
- The == tests value equivalence
Python recursively tests nested comparisons until a result is ascertained.
- The
is
operator tests object identity
Python tests whether the two are really the same object and live at the same address in memory.
So let’s compare some strings, shall we?
StringThing1 = "water"
StringThing2 = "water"
StringThing1 == StringThing2, StringThing1 is StringThing2
(True, True)
Ok, what just happened? We need to be very careful here and i have seen this cause some really ugly bugs when performing long-chained regex stuff with health data. Python internally caches and reuses some strings as an optimization technique. Here there is really just a single string ‘water’ in memory shared by S1, S2
thus the identity operator evaluates to True
.
The workaround is thus:
StringThing1 = "i wish you water"
StringThing2 = "i wish you water"
StringThing1 == StringThing2,StringThing1 is StringThing2
(True, False)
Given the logic of this lets see how we have conditional logic comparisons.
I believe Python 2.5 introduced ternary operators. Once again interesting word:
Ternary operators ternary means composed of three parts or three as a base.
The operators are the fabled if/else
you see in almost all programming languages.
Whentrue if
condition else
whenfalse
The condition is evaluated first. If condition is true the result is whentrue; otherwise the result is whenfalse. Only one of the two subexpressions whentrue and whenfalse evaluates depending on the truth value of condition.
Stylistically you want to palace parentheses around the whole expression.
Example of operator this was taken directly out the Python docs with a slight change as i thought it was funny:
is_nice = True
state = "nice" if is_nice else "ain’t nice"
print(state)
Which also shows how Python treats True and False.
In most programming languages an integer 0 is FALSE and an integer 1 is TRUE.
However, Python looks at an empty data structure as False. True and False as illustrated above are inherent properties of every object in Python.
So in general Python compares types as follows:
- Numbers are compared by the relative magnitude
- Non-numeric mixed types comparisons where
( 3 < ‘water’)
doesn’t fly in Python 3.0 However they are allowed in Python 2.6 where they use a fixed arbitrary rule. Same with sorts non-numeric mixed type collections cannot be sorted in Python 3.0 - Strings are compared lexicographically (ok cool word what does it mean?). Iin mathematics, the lexicographic or lexicographical order is a generalization of the alphabetical order of the dictionaries to sequences of ordered symbols or, more generally, of elements of a totally ordered set. In other words like a dictionary. Character by character where
(“abc” < “ac”)
- Lists and tuples are compared component by component left to right
- Dictionaries are compared as equal if their sorted
(key, value)
lists are equal. However relative magnitude comparisons are not supported in Python 3.0
With structured objects as one would think the comparison happens as though you had written the objects as literal and compared all the components one at a time left to right.
Further, you can chain the comparisons such as:
a < b <= c < d
Which functionally is the same thing as:
a < b and b <= c and c < d
The chain form is more compact and more readable and evaluates each subexpression once at the most.
Being that most reading this should be using Python 3.0 a couple of words on dictionaries per the last commentary. In Python 2.6 dictionaries supported magnitude comparisons as though you were comparing (key,value)
lists.
In Python 3.0 magnitude comparisons for dictionaries are removed because they incur too much overhead when performing equality computations. Python 3.0 from what i can gather uses an in-optimized scheme for equality comparisons. So you write loops or compare them manually. Once again no free lunch. The documentation can be found here: Ordering Comparisons in Python 3.0.
One last thing. There is a special object called None
. It’s a special data type in Python in fact i think the only special data type. None
is equivalent to a Null
pointer in C.
This comes in handy if your list size is not known:
MyList = [None] * 50
Print (MyList)
[None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None, None]
The output makes me think of a Monty Python skit. See what I did there? While the comparison to a NULL pointer is correct the way in which it allocates memory and doesn’t limit the size of the list it allocates presets an initial size to allow for future indexing assignments. In this way, it kind of reminds me of malloc
in C. Purist please don’t shoot the messenger.
Well, i got a little long in the tooth as they say. See what i did again? Teeth, Snakes and Python.
See y’all next week.
Until Then,
Muzak To Blog By: various tunes by : Pink Martini, Pixies, Steve Miller.