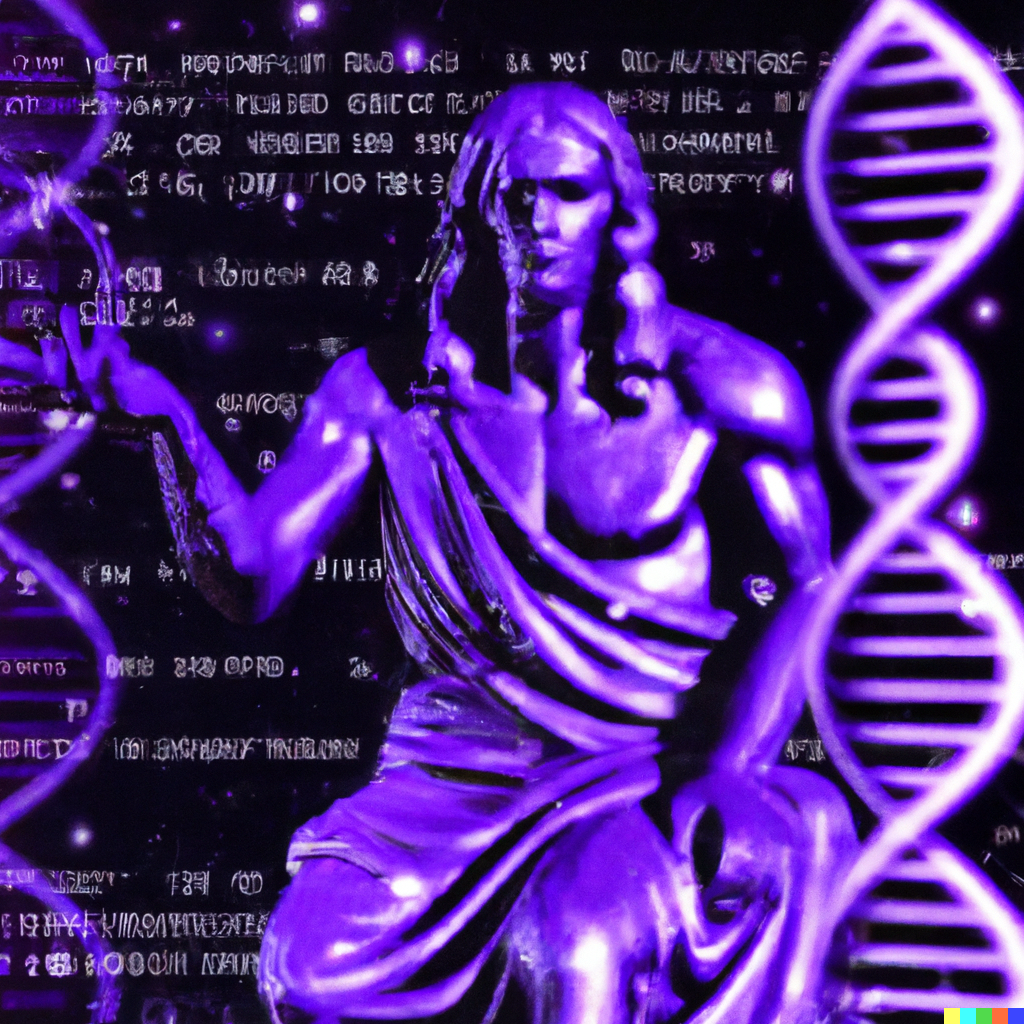
Your vision will only become clear when you can look into your heart. Who looks outside, dreams; who looks inside, awakes. Knowing your own darkness is the best method for dealing with the darknesses of other people. We cannot change anything until we accept it.
~ C. Jung
(Caveat Emptor: This blog is rather long in the snakes tooth and actually more like a CHOMP instead of a BYTE. tl;dr)
First, Oh Dear Reader i trust everyone is safe, Second sure feels like we are living in an age of Deus Ex Machina, doesn’t it? Third with this in mind i wanted to write a Snake_Byte
that have been “thoughting” about for quite some but never really knew how to approach it if truth be told. I cant take full credit for this ideation nor do i actually want to claim any ideation. Jay Sales and i were talking a long time after i believe i gave a presentation on creating Belief Systems using BeliefNetworks or some such nonsense.
The net of the discussion was we both believed that in the future we will code in philosophical frameworks.
Maybe we are here?
So how would one go about coding an agent-based distributed system that allowed one to create an agent or a piece of evolutionary code to exhibit said behaviors of a philosophical framework?
Well we must first attempt to define a philosophy and ensconce it into a quantized explanation.
Stoicism seemed to me at least the best first mover here as it appeared to be the tersest by definition.
So first those not familiar with said philosophy, Marcus Aurelius was probably the most famous practitioner of Stoicism. i have put some references that i have read at the end of this blog1.
Stoicism is a philosophical school that emphasizes rationality, self-control, and inner peace in the face of adversity. In thinking about this i figure To build an agent-based software system that embodies Stoicism, we would need to consider several key aspects of this philosophy.
- Stoics believe in living in accordance with nature and the natural order of things. This could be represented in an agent-based system through a set of rules or constraints that guide the behavior of the agents, encouraging them to act in a way that is in harmony with their environment and circumstances.
- Stoics believe in the importance of self-control and emotional regulation. This could be represented in an agent-based system through the use of decision-making algorithms that take into account the agent’s emotional state and prioritize rational, level-headed responses to stimuli.
- Stoics believe in the concept of the “inner citadel,” or the idea that the mind is the only thing we truly have control over. This could be represented in an agent-based system through a focus on internal states and self-reflection, encouraging agents to take responsibility for their own thoughts and feelings and strive to cultivate a sense of inner calm and balance.
- Stoics believe in the importance of living a virtuous life and acting with moral purpose. This could be represented in an agent-based system through the use of reward structures and incentives that encourage agents to act in accordance with Stoic values such as courage, wisdom, and justice.
So given a definition of Stoicism we then need to create a quantized model or discrete model of those behaviors that encompass a “Stoic Individual”. i figured we could use the evolutionary library called DEAP (Distributed Evolutionary Algorithms in Python ). DEAP
contains both genetic algorithms and genetic programs utilities as well as evolutionary strategy methods for this type of programming.
Genetic algorithms and genetic programming are both techniques used in artificial intelligence and optimization, but they have some key differences.
This is important as people confuse the two.
Genetic algorithms are a type of optimization algorithm that use principles of natural selection to find the best solution to a problem. In a genetic algorithm, a population of potential solutions is generated and then evaluated based on their fitness. The fittest solutions are then selected for reproduction, and their genetic information is combined to create new offspring solutions. This process of selection and reproduction continues until a satisfactory solution is found.
On the other hand, genetic programming is a form of machine learning that involves the use of genetic algorithms to automatically create computer programs. Instead of searching for a single solution to a problem, genetic programming evolves a population of computer programs, which are represented as strings of code. The programs are evaluated based on their ability to solve a specific task, and the most successful programs are selected for reproduction, combining their genetic material to create new programs. This process continues until a program is evolved that solves the problem to a satisfactory level.
So the key difference between genetic algorithms and genetic programming is that genetic algorithms search for a solution to a specific problem, while genetic programming searches for a computer program that can solve the problem. Genetic programming is therefore a more general approach, as it can be used to solve a wide range of problems, but it can also be more computationally intensive due to the complexity of evolving computer programs2.
So returning back to the main() function as it were, we need create a genetic program that models Stoic behavior using the DEAP library,
First need to define the problem and the relevant fitness function. This is where the quantized part comes into play. Since Stoic behavior involves a combination of rationality, self-control, and moral purpose, we could define a fitness function that measures an individual’s ability to balance these traits and act in accordance with Stoic values.
So lets get to the code.
To create a genetic program that models Stoic behavior using the DEAP
library in a Jupyter Notebook, we first need to install the DEAP
library. We can do this by running the following command in a code cell:
pip install deap
Next, we can import the necessary modules and functions:
import random import operator import numpy as np from deap import algorithms, base, creator, tools
We can then define the problem and the relevant fitness function. Since Stoic behavior involves a combination of rationality, self-control, and moral purpose, we could define a fitness function that measures an individual’s ability to balance these traits and act in accordance with Stoic values.
Here’s an example of how we might define a “fitness function” for this problem:
#Define the fitness function. NOTE:
# i am open to other ways of defining this and other models # the definition of what is a behavior needs to be quantized or discretized and # trying to do that yields a lossy functions most times. Its also self referential def fitness_function(individual): # Calculate the fitness based on how closely the individual's behavior matches stoic principles fitness = 0 # Add points for self-control, rationality, focus, resilience, and adaptability can haz Stoic? fitness += individual[0] # self-control fitness += individual[1] # rationality fitness += individual[2] # focus fitness += individual[3] # resilience fitness += individual[4] # adaptability return fitness, # Define the genetic programming problem creator.create("FitnessMax", base.Fitness, weights=(1.0,)) creator.create("Individual", list, fitness=creator.FitnessMax) # Initialize the genetic algorithm toolbox toolbox = base.Toolbox() # Define the genetic operators toolbox.register("attribute", random.uniform, 0, 1) toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attribute, n=5) toolbox.register("population", tools.initRepeat, list, toolbox.individual) toolbox.register("evaluate", fitness_function) toolbox.register("mate", tools.cxTwoPoint) toolbox.register("mutate", tools.mutGaussian, mu=0, sigma=0.1, indpb=0.1) toolbox.register("select", tools.selTournament, tournsize=3) # Run the genetic algorithm population = toolbox.population(n=10) for generation in range(20): offspring = algorithms.varAnd(population, toolbox, cxpb=0.5, mutpb=0.1) fits = toolbox.map(toolbox.evaluate, offspring) for fit, ind in zip(fits, offspring): ind.fitness.values = fit population = toolbox.select(offspring, k=len(population)) # Print the best individual found best_individual = tools.selBest(population, k=1)[0] print ("Best Individual:", best_individual)
Here, we define the genetic programming parameters (i.e., the traits that we’re optimizing for) using the toolbox.register
function. We also define the evaluation function (stoic_fitness)
, genetic operators (mate and mutate), and selection operator (select) using DEAP’s built-in functions.
We then define the fitness function that the genetic algorithm will optimize. This function takes an “individual” (represented as a list of five attributes) as input, and calculates the fitness based on how closely the individual’s behavior matches stoic principles.
We then define the genetic programming problem via the quantized attributes, and initialize the genetic algorithm toolbox with the necessary genetic operators.
Finally, we run the genetic algorithm for 20 generations, and print the best individual found. The selBest
function is used to select the top individual fitness agent or a “behavior” if you will for that generation based on the iterations or epochs. This individual represents an agent that mimics the philosophy of stoicism in software, with behavior that is self-controlled, rational, focused, resilient, and adaptable.
Best Individual: [0.8150247518866958, 0.9678037028949047, 0.8844195735244268, 0.3970642186025506, 1.2091810770505023]
This denotes the best individual with those best balanced attributes or in this case the Most Stoic,
As i noted this is a first attempt at this problem i think there is a better way with a full GP solution as well as a tunable fitness function. In a larger distributed system you would then use this agent as a framework amongst other agents you would define.
i at least got this out of my head.
until then,
#iwishyouwater <- Alexey Molchanov and Dan Bilzerian at Deep Dive Dubai
Muzak To Blog By: Phil Lynott “The Philip Lynott Album”, if you dont know who this is there is a statue in Ireland of him that i walked a long way with my co-founder, Lisa Maki a long time ago to pay homage to the great Irish singer of the amazing band Thin Lizzy. Alas they took Phil to be cleaned that day. At least we got to walk and talk and i’ll never forget that day. This is one of his solo efforts and i believe he is one of the best artists of all time. The first track is deeply emotional.
References:
[1] A list of books on Stoicism -> click HERE.
[2] Genetic Programming (On the Programming of Computers by Means of Natural Selection), By Professor John R. Koza. There are multiple volumes i think four and i have all of this but this is a great place to start and the DEAP documentation. Just optimizing a transcendental functions is mind blowing what GP comes out with using arithmetic